Python - Hackerrank String Object Manipulation Program
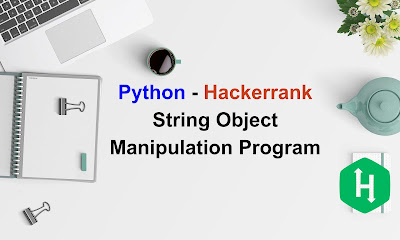 |
Python - Hackerrank String Object Manipulation Program |
Source Code
Aim :
To program a Hackerrank string object manipulation code in python.
Algorithm :
- Get the required input from the user by using input statement for 'q'(number of vehicle) and 'args'(Type of vehicle with respective speed limit).
- Instantiation the Car() and Boat() class and their function with passing parameter as 'args'.
- Write the required __str__ function definition for the class Car() and Boat().
- The required information is successfully updated in the given file (junk.txt).
Program :
#!/bin/python3
import math
import os
import random
import re
import sys
class Car:
def __init__(self, max_speed, speed_unit):
self.speed = max_speed
self.unit = speed_unit
def __str__(self):
return "Car with the maximum speed of {} {}".format(self.speed, self.unit)
class Boat:
def __init__(self, max_speed):
self.speed = max_speed
def __str__(self):
return "Boat with the maximum speed of {} knots".format(self.speed)
if __name__ == '__main__':
os.environ['OUTPUT_PATH'] = 'junk.txt'
fptr = open(os.environ['OUTPUT_PATH'], 'w')
q = int(input("Enter the number of vehicle : "))
queries = []
for _ in range(q):
args = input("Enter vehicle with respective speed unit : ").split()
vehicle_type, params = args[0], args[1:]
if vehicle_type == "car":
max_speed, speed_unit = int(params[0]), params[1]
vehicle = Car(max_speed, speed_unit)
elif vehicle_type == "boat":
max_speed = int(params[0])
vehicle = Boat(max_speed)
else:
raise ValueError("invalid vehicle type")
fptr.write("%s\n" % vehicle)
fptr.close()
Output :
Enter the number of vehicle :
2
Enter vehicle with respective speed unit :
car 100 kmph
Enter vehicle with respective speed unit :
boat 82
Text That Appear On A Given File Are Below :
Car with the maximum speed of 100 kmph
Boat with the maximum speed of 82 knots
Terminal Code :
 |
Python - Hackerrank String Object Manipulation Program01
|
 |
Python - Hackerrank String Object Manipulation Program02 |
Terminal Output :
 |
Python - Hackerrank String Object Manipulation Output |
Result :
The output was executed successfully and result was verified.
Personal Writings for Readers!!!
Story behind single family described in Pandemic Family Book.
Read more on...
Paperback - https://amzn.to/34NS6Sk
Amazon Kindle - https://amzn.to/3aKtIng
Kobo Writing - https://bit.ly/3iQysNy
Comments
Post a Comment